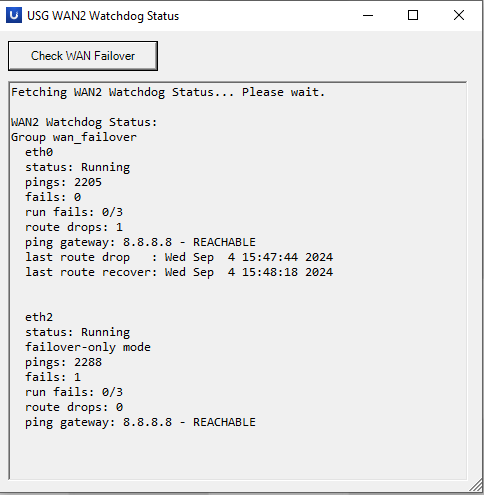
Unifi Security Gateway - PowerShell GUI to check Failover
I was having internet issues, and my UniFi Security Gateway would failover to the backup 4G internet connection. When this happens, I would notice (slower connection, and at the time a small blip) and then I would log in via SSH to the USG and check the watchdog status for the failover connection.
Using the joys of AI and PowerShell I (and claude.ai) have made a small PowerShell GUI Application to login via SSH and output the command show load-balance watchdog.
Click the button, find out. Quick and easy. It even gets a icon from the ui.com website for the logo in the top left :)
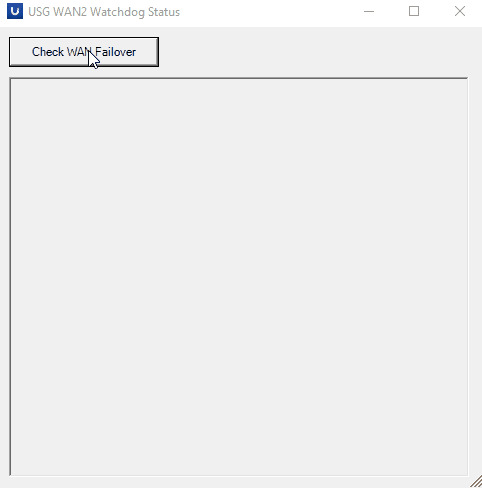
Add-Type -AssemblyName System.Windows.Forms
Add-Type -AssemblyName System.Drawing
# Function to download and save the Ubiquiti icon
function Get-UbiquitiIcon {
$iconUrl = "https://ui.com/favicon.ico"
$iconPath = Join-Path $env:TEMP "ubiquiti_icon.ico"
try {
Invoke-WebRequest -Uri $iconUrl -OutFile $iconPath
return $iconPath
}
catch {
Write-Warning "Failed to download Ubiquiti icon: $_"
return $null
}
}
# Download the Ubiquiti icon
$iconPath = Get-UbiquitiIcon
# Create the main form
$form = New-Object System.Windows.Forms.Form
$form.Text = "USG WAN2 Watchdog Status"
$form.Size = New-Object System.Drawing.Size(500,500)
$form.StartPosition = "CenterScreen"
# Set the form icon if download was successful
if ($iconPath -and (Test-Path $iconPath)) {
$form.Icon = [System.Drawing.Icon]::ExtractAssociatedIcon($iconPath)
}
# Create a button
$button = New-Object System.Windows.Forms.Button
$button.Location = New-Object System.Drawing.Point(10,10)
$button.Size = New-Object System.Drawing.Size(150,30)
$button.Text = "Check WAN Failover"
$form.Controls.Add($button)
# Create a rich text box to display results
$outputBox = New-Object System.Windows.Forms.RichTextBox
$outputBox.Location = New-Object System.Drawing.Point(10,50)
$outputBox.Size = New-Object System.Drawing.Size(460,400)
$outputBox.Font = New-Object System.Drawing.Font("Consolas", 10)
$outputBox.ReadOnly = $true
$outputBox.WordWrap = $false
$outputBox.ScrollBars = "Both"
$form.Controls.Add($outputBox)
# Function to run SSH command using plink
function Invoke-SshCommand {
param (
[string]$command
)
$plinkPath = "C:\Program Files\PuTTY\plink.exe" # Update this path if necessary
$usgIpAddress = "10.11.12.1" # Update with your USG IP
$sshUsername = "administrator" # Update with your SSH username
$sshPassword = "your_password_here" # Update with your SSH password
$sshOutput = $null
try {
$plinkCommand = "echo y | & `"$plinkPath`" -ssh $usgIpAddress -l $sshUsername -pw $sshPassword `"/opt/vyatta/bin/vyatta-op-cmd-wrapper $command`""
$sshOutput = Invoke-Expression $plinkCommand
}
catch {
$sshOutput = "Error executing SSH command: $_"
}
return $sshOutput
}
# Button click event handler
$button.Add_Click({
$outputBox.Clear()
$outputBox.AppendText("Fetching WAN2 Watchdog Status... Please wait.`r`n`r`n")
$form.Refresh()
$watchdogStatus = Invoke-SshCommand -command "show load-balance watchdog"
if ($watchdogStatus) {
$outputBox.AppendText("WAN2 Watchdog Status:`r`n")
# Split the output into lines and append each line separately
$watchdogStatus -split "`n" | ForEach-Object {
$outputBox.AppendText("$_`r`n")
}
}
else {
$outputBox.AppendText("Failed to retrieve WAN2 watchdog status.")
}
})
# Show the form
$form.ShowDialog()
# Clean up the temporary icon file
if ($iconPath -and (Test-Path $iconPath)) {
Remove-Item $iconPath -Force
}
Then create a shortcut to powershell.exe "C:\Neil\USG-WatchDog-GUI.ps1" and its all done.
Please Leave Comments